Auth Webhook
Overview
An Auth Webhook enables fine-grained access control for your application by validating client requests through an external authentication server. This mechanism allows you to implement custom authorization logic, ensuring that only authorized users can perform specific operations on Yorkie documents.
(5) Response (4) Handle request┌─────────────────┐ ┌──┐│ │ │ │ (3) Response▼ │ ▼ │ - allowed┌──────┐ ┌┴─────┤ - reason ┌───────────────┐│Client├────────────►│Server│◄──────────────┤External Server│└──────┘ (1)Request └────┬─┘ └───────────────┘- token │ ▲- dockey └──────────────────────┘(2) Call webhook- token- dockey- verb: r or rw
The workflow follows these steps:
- Client makes a request with an authentication token
- Yorkie server forwards the request details to your webhook server
- Your webhook server validates the request and returns a response
- Based on the webhook response, Yorkie server either processes or rejects the original request
Setup and Configuration
1. Configure the Auth Webhook
The simplest way to set up Auth Webhook is through the Dashboard:
- Navigate to the Project Settings page in the Dashboard
- Configure the webhook URL and specify the methods to be authenticated
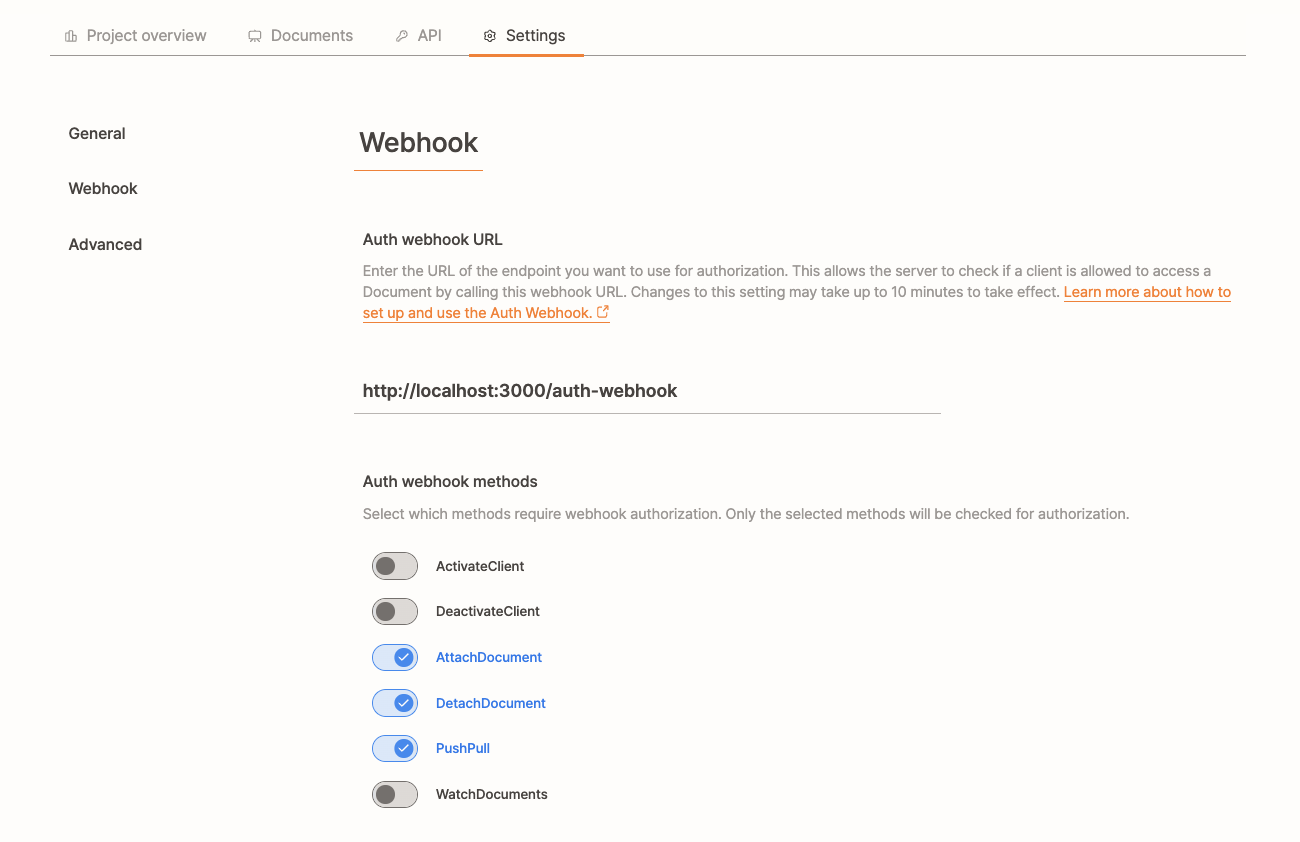
You can also use the Yorkie CLI. Refer to the updating the project.
- Configuration changes may take up to 10 minutes to take effect.
- If an Auth Webhook URL is set without specifying methods, authentication will be performed for ALL methods.
- To disable authentication completely, you must remove the Auth Webhook URL.
2. Client Configuration
Use the authTokenInjector
option when creating a client to provide authentication tokens:
1const client = new yorkie.Client('https://api.yorkie.dev', {2 authTokenInjector: async (reason) => {3 // Handle token refresh logic based on webhook response4 if (reason === 'token expired') {5 return await refreshAccessToken();6 }7 return accessToken;8 },9});
The authTokenInjector will be called again with the webhook's response reason if a codes.Unauthenticated
error occurs, allowing the system to refresh tokens.
3. Webhook Request Format
When a client makes a request, the server sends the following information to your webhook server:
{"token": "SOME_TOKEN", // Token from authTokenInjector"method": "PushPull", // Method being called: ActivateClient, DeactivateClient, AttachDocument, DetachDocument, WatchDocuments"documentAttributes": [{"key": "DOCUMENT_KEY", // Document key"verb": "r" // Access level: 'r' or 'rw'}]}
4. Webhook Response Format
Your webhook server should respond with:
{"allowed": true, // Authorization decision"reason": "ok" // Optional explanation}
HTTP Status Codes:
200 OK
: Request is authorized401 Unauthenticated
: Token is invalid or missing403 Permission Denied
: Token is valid but lacks required permissions
5. Error Handling and Recovery
- Successful Authorization (200 + allowed:true)
- Request proceeds normally
- No special handling required
- Permission Denied (403 + allowed:false)
- Request fails with
codes.PermissionDenied
- Request fails with
- Unauthenticated (401 + allowed:false)
- System attempts token refresh via
authTokenInjector
- For
PushPull
in realtime sync mode andWatchDocuments
:- Automatically retries after token refresh
- Notifies via
document.subscribe('auth-error')
- For Other Methods:
- Requires manual retry when handling
codes.Unauthenticated
error
- Requires manual retry when handling
- System attempts token refresh via